Performing Sentiment Analysis Using Earnings Call Data
Introduction to Sentiment Analysis
Sentiment analysis, also known as opinion mining, is a natural language processing (NLP) technique used to determine the sentiment expressed in a piece of text. It classifies the text into categories such as positive, negative, or neutral. This technique is widely used in various fields to gauge public opinion, monitor brand reputation, and understand customer feedback.
Examples of Sentiment Analysis
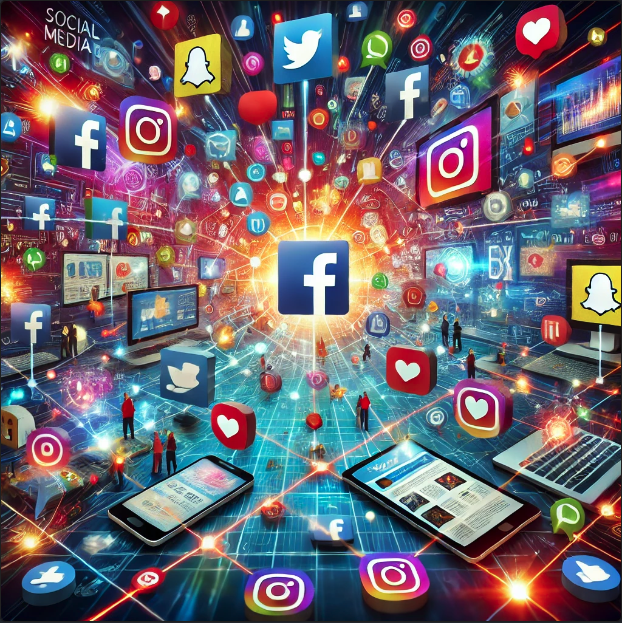
- Social Media Monitoring: Companies use sentiment analysis to track mentions of their brands on social media platforms. By analyzing the sentiment of these mentions, they can understand public perception and address any issues promptly.
- Customer Reviews: Businesses analyze customer reviews to gain insights into their products or services. Positive reviews highlight strengths, while negative reviews point out areas for improvement.
- Market Research: Analysts use sentiment analysis to assess public opinion on market trends, political events, or product launches. This helps in making informed decisions based on public sentiment.
Earnings Call API
Earnings calls are a vital source of information for investors and analysts. These calls provide insights into a company’s performance, future prospects, and management’s outlook. By performing sentiment analysis on earnings call transcripts, investors can gauge the sentiment expressed by the company’s executives and make more informed investment decisions.
We will use the Earnings call Python Library to retrieve earnings conference call transcripts, and then to perform sentiment analysis on each one.
Installing the Required Dependencies
First, ensure you are running at least Python 3.8
or greater. Next, we need to install the required python packages.
We will be using TextBlob Python Library to perform sentiment analysis on the earnings call transcripts.
pip install --upgrade earningscall textblob matplotlib
Next, we will use the earningscall
library to retrieve a single transcript. We'll use Apple's 1st Quarter 2024 earnings conference call.
from earningscall import get_company
company = get_company("AAPL")
transcript = company.get_transcript(quarter=1, year=2024) # Fetch the transcript for Q1, 2024
print(f"Transcript: \"{transcript.text[:100]}\"")
Output:
Transcript: "Good day, and welcome to the Apple Q1 Fiscal Year 2024 Earnings Conference Call. Today's call is bei"
Now, we use TextBlob
to perform sentiment analysis on the entire text:
from textblob import TextBlob
# Perform sentiment analysis on the transcript
blob = TextBlob(transcript.text)
print(f"Sentiment Polarity: {blob.sentiment.polarity}")
print(f"Sentiment Subjectivity: {blob.sentiment.subjectivity}")
Output:
Sentiment Polarity: 0.18496337217726486
Sentiment Subjectivity: 0.46769073323131016
The Docs for sentiment object are as follows:
Return a tuple of form (polarity, subjectivity ) where polarity
is a float within the range [-1.0, 1.0] and subjectivity is a float
within the range [0.0, 1.0] where 0.0 is very objective and 1.0 is
very subjective.
So, for Apple's Q1 2024 call, the sentiment is slightly positive (0.18
) and moderately subjective (0.47
).
Sentiment Analysis on S&P 500 Companies
Performing sentiment analysis on a single conference call is great, but let's use the power of data to perform this same task on all 500 companies in the S&P 500!
In order to gain access to Earnings Call Data for the rest of the S&P500 companies, you'll need an EarningsCall API Key, which you can get from this page. You can configure the API Key like this:
import earningscall
earningscall.api_key = "YOUR SECRET API KEY GOES HERE"
Next, let's define a function to generate Polarity and Subjectivity for all earnings call transcripts for a given Company. We'll use the following code:
from typing import Iterator
from earningscall.company import Company
def get_datapoints(company: Company) -> Iterator[list]:
for event in company.events():
transcript = company.get_transcript(event=event) # Fetch the earnings call transcript for this event
if not transcript:
continue
blob = TextBlob(transcript.text)
sentiment = blob.sentiment
x = sentiment.polarity
y = sentiment.subjectivity
yield [x, y]
Now, we can define a function to loop over all companies in the S&P 500, generating data points for all of these companies:
from earningscall import get_sp500_companies
def get_all_datapoints(n: int = 50):
i = 0
for company in get_sp500_companies():
i += 1
for datapoint in get_datapoints(company):
yield datapoint
if i >= n:
break
Finally, we can generate the datapoints by calling the function, then converting the datapoints into x
and y
arrays. NOTE: this takes quite a while to complete (roughly 10 minutes).
x, y = list(zip(*list(get_all_datapoints(n=500))))
Now that we have our x
and y
arrays, we can use them to produce a scatter plot.
from matplotlib import pyplot as plt
fig, ax = plt.subplots()
# Map each onto a scatterplot we'll create with Matplotlib
ax.scatter(x=x, y=y, s=0.5)
ax.set(title="Sentiment of EarningsCall Transcripts",
xlabel='Polarity',
ylabel='Subjectivity')
plt.show()
Output:
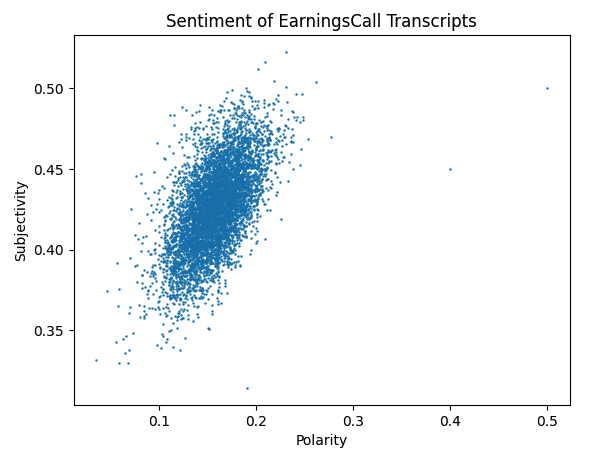
As you can see, most of the transcripts polarity fell between 0.1
and 0.25
. Interestingly, no transcript fell into negative territory – they we all greater than zero. Furthermore, there seems to be correlation between the polarity and the subjectivity of the transcript – the greater the polarity the greater the subjectivity. There were a couple of outliers that fell into the greater than 0.4
polarity category.
For the full code in this article, please see the following:
Summary
We provided some simple Python code using the EarningsCall and TextBlob libraries to perform sentiment analysis on Earnings Call Transcripts. We used the matplotlib
library to create a scatter plot of the sentiment (Polarity and Subjectivity) of the earnings call conference transcripts from all S&P 500 companies.